How to build Defi App?
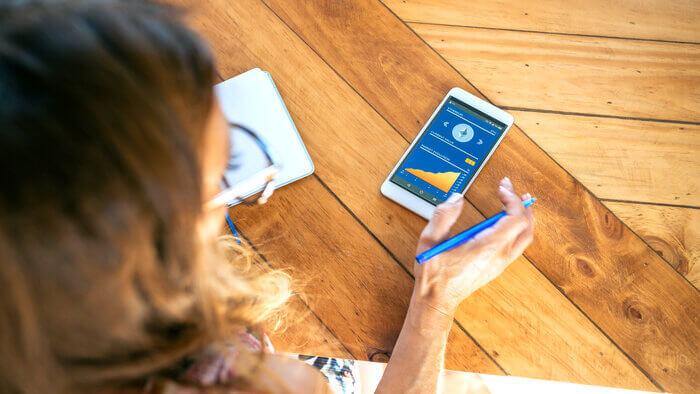
The advent of decentralized finance within the crypto space has created a lot of traction worldwide. Defi is growing fast would be an understatement. With its introduction, it started to gain immense popularity among tech enthusiasts somewhere around mid of 2018. By 2019, Defi associated itself with over half a billion dollars market valueIn today’s market, the value of Defi has now risen over $26 billion.
As Defi apps’ demands grow, learning the particular field’s implementation skill set and knowledge also increases. This article focuses on providing a step-by-step approach to develop a Defi app using Solidity. Through this Defi application, users can deposit an ERC20 token to the smart contract and it’ll mint and transfer Farm Tokens to them. When users want to withdraw their ERC20 tokens, they can burn their Farm tokens and then ERC 20 tokens are transferred back to them.
The article moves towards the description of creating a Defi app after a brief introduction about Defi applications.
What are Defi apps?
Defi applications built on a Blockchain network provide all financial services in a decentralized, borderless manner that allows everyone to access and avail all financial services. Defi applications allow lending and borrowing funds, trade cryptocurrencies, speculate the price movements, earn interests and provide risk insurance. Transactions made through centralized intermediaries are now performed directly between participants, mediated by smart contracts.
How to build Defi apps?
An environment setup is required to start working on the development of the application. Two tools, Truffle and Ganache, require installation.
Comprehensive development services to help you lead the future-ready DeFi projects.
Launch your DeFi project with LeewayHertz
1. Install Truffle and Ganache
Truffle is a testing and development framework for building smart contracts for Ethereum. It provides an easy way to create and deploy smart contracts to the blockchain. The other tool, Ganache, allows creating local Ethereum blockchain to test smart contracts. Ganache simulates basic network features and funds the first ten accounts with 100 test Ether. Thus, it makes the deployment of Smart contracts free and easy. It can be used as a desktop application or command-line tool. Here, a UI desktop application is used.
Project creation involves executing the following commands:
It will create a blank project and the structure of the project will be as shown below:
- contracts: Folder for the Solidity Smart contracts
- migrations: Folder for the deployment scripts
- test: Folder for testing our Smart contracts
- truffle-config.js: Truffle configuration file
2. Create an ERC20 token
ERC20 is a fungible token that is used to stake on the smart contract. To create this token, the OpenZeppelin library is necessary to install. This library consists of implementation standards for ERC20 and ERC721. Its installation requires to run following command:
Using the library OpenZeppelin, an ERC20 token called MyToken is created with implementing the following code:
In this code:
- Line 3 imports the contract ERC20.sol from the installed library.
- Line 6 calls ERC20.sol constructor and passes for the name and symbol parameters as “MyToken” and “MTKN,” respectively.
- Line 7 is minting and transferring 1 million tokens for the account that is deploying the smart contract.
ERC20.sol constructor implementation where the “_decimals” field is set to 18 is shown below:
3. Deploy ERC20 token
Create a file named 2_deploy_Tokens.js on the migrations folder. It will deploy both the ERC20 token and the FarmToken smart contract. To deploy MyToken.sol contract:
Before the compilation of the Smart contract, first, check the Solidity compiler version.
Solidity’s default version is v0.5.16. The token here is written in version 0.6.2. If commands are executed to compile the contract, it will generate a compiler error. Therefore, it is necessary to check the version. To specify the Solidity compiler version, go to truffleconfig.js file and set the desired compiler version as shown below:
Compile the smart contract with the following command:
Now, after compilation, move forward to deploy the token. Open Ganache and go to the “Quickstart” option. It will start local Ethereum Blockchain. To deploy the contract, execute the following:
Truffle console verifies that 1 million MyToken tokens have been sent to the deployer address. To interact with the smart contract, run:
Now, write the following command:
-
- For smart contract:
-
- From Ganache, get the array of accounts:
-
- To check the balance:
-
- To format the balance from 18 decimals:
4. Create Farm Token Smart Contract
A FarmToken smart contract performs three main functions:
-
- deposit(uint256 _amount): On behalf of the user, transfer MyToken to FarmToken smart contract. Then, mint and transfer the FarmToken to the user.
- withdraw(uint256 _amount): Burns user’s FarmToken and transfer MyToken to its address.
- balance(): Get MyToken balance on FarmToken smart contract. For FarmToken constructor:
- Lines 3-6 import the following contract from OpenZeppelin
IERC20.sol, Address.sol, SafeERC20.sol and ERC20.sol. - Line 8 represents the inheritance of FarmToken from the ERC20 contract.
- Line 14-19 enables the FarmToken constructor to receive the MyToken contract’s address as a parameter and assign it to a public variable called the token.
Implement balance() function:
For deposit() function:
To implement withdraw() function:
Now, deploy smart contract:
Remember that while deploying the FarmToken, the MyToken smart contract’s address is passed as a parameter. Run truffle migrate and truffle compiler to deploy the contracts. Rather than using the truffle console to interact with the smart contract, create a script to automate the process. Create a “scripts” folder and add the “getMyTokenBalance.js” file. It’ll check the balance of MyToken in FarmToken smart contract.
Run the following CLI command to execute this script:
truffle exec .\scripts\getMyTokenBalance.js
Comprehensive development services to help you lead the future-ready DeFi projects.
Launch your DeFi project with LeewayHertz
Here, the expected result is 0. Now, MyToken is to be staked on the smart contract. The user needs to first approve the smart contract to transfer MyToken, since the deposit(uint256 _amount) function calls safeTransfer() function. To approve the request call the function:
const MyToken = artifacts.require(“MyToken”); const FarmToken = artifacts.require(“FarmToken”); module.exports = async function(callback) { const accounts = await new web3.eth.getAccounts(); const myToken = await MyToken.deployed(); const farmToken = await FarmToken.deployed(); const allowanceBefore = await myToken.allowance(accounts[0], farmToken.address); console.log(‘Amount of MyToken FarmToken is allowed to transfer on our behalf Before: ‘ + allowanceBefore.toString()); await myToken.approve(farmToken.address, web3.utils.toWei(‘100’, ‘ether’)); const allowanceAfter = await myToken.allowance(accounts[0], farmToken.address); console.log(‘Amount of MyToken FarmToken is allowed to transfer on our behalf After: ‘ + allowanceAfter.toString()); balanceMyTokenBeforeAccounts0 = await myToken.balanceOf(accounts[0]); balanceMyTokenBeforeFarmToken = await myToken.balanceOf(farmToken.address); console.log(‘*** My Token ***’) console.log(‘Balance MyToken Before accounts[0] ‘ + web3.utils.fromWei(balanceMyTokenBeforeAccounts0.toString())) console.log(‘Balance MyToken Before TokenFarm ‘ + web3.utils.fromWei(balanceMyTokenBeforeFarmToken.toString())) console.log(‘*** Farm Token ***’) balanceFarmTokenBeforeAccounts0 = await farmToken.balanceOf(accounts[0]); balanceFarmTokenBeforeFarmToken = await farmToken.balanceOf(farmToken.address); console.log(‘Balance FarmToken Before accounts[0] ‘ + web3.utils.fromWei(balanceFarmTokenBeforeAccounts0.toString())) console.log(‘Balance FarmToken Before TokenFarm ‘ + web3.utils.fromWei(balanceFarmTokenBeforeFarmToken.toString())) console.log(‘Call Deposit Function’) await farmToken.deposit(web3.utils.toWei(‘100’, ‘ether’)); console.log(‘*** My Token ***’) balanceMyTokenAfterAccounts0 = await myToken.balanceOf(accounts[0]); balanceMyTokenAfterFarmToken = await myToken.balanceOf(farmToken.address); console.log(‘Balance MyToken After accounts[0] ‘ + web3.utils.fromWei(balanceMyTokenAfterAccounts0.toString())) console.log(‘Balance MyToken After TokenFarm ‘ + web3.utils.fromWei(balanceMyTokenAfterFarmToken.toString())) console.log(‘*** Farm Token ***’) balanceFarmTokenAfterAccounts0 = await farmToken.balanceOf(accounts[0]); balanceFarmTokenAfterFarmToken = await farmToken.balanceOf(farmToken.address); console.log(‘Balance FarmToken After accounts[0] ‘ + web3.utils.fromWei(balanceFarmTokenAfterAccounts0.toString())) console.log(‘Balance FarmToken After TokenFarm ‘ + web3.utils.fromWei(balanceFarmTokenAfterFarmToken.toString())) callback(); }
Run this script with:
truffle exec .\scripts\transferMyTokenToFarmToken.js
Now, the job to successfully create and deploy a defi app is done.
Conclusion
The article presented how to set up the project development environment with Truffle and Ganache and create a Defi application that can deposit MyTokens, receive FarmTokens and withdraw MyTokens by burning FarmTokens.
If you’re interested and looking for a company to develop a Defi application for your business, we’re ready to do it for you. Get a consultation scheduled with our team of experts and discuss your requirements.
Start a conversation by filling the form
All information will be kept confidential.
Insights
AI agents for due diligence: Role, use cases and applications, benefits, and implementation
AI agents in due diligence are intelligent systems designed to assist professionals in various industries by automating due diligence tasks, analyzing data, and generating insights.
AI for financial reporting: Use cases and applications, architecture, benefits, implementation, best practices and future trends
AI is significantly transforming financial reporting by automating routine tasks, enhancing accuracy, and providing real-time insights.
AI agents in compliance: Role, use cases and applications, benefits, and implementation
Adopting AI agents within compliance practices offers many transformative advantages, streamlining processes and enhancing the efficacy of regulatory practices.