Steps to Create, Test and Deploy Ethereum Smart Contract
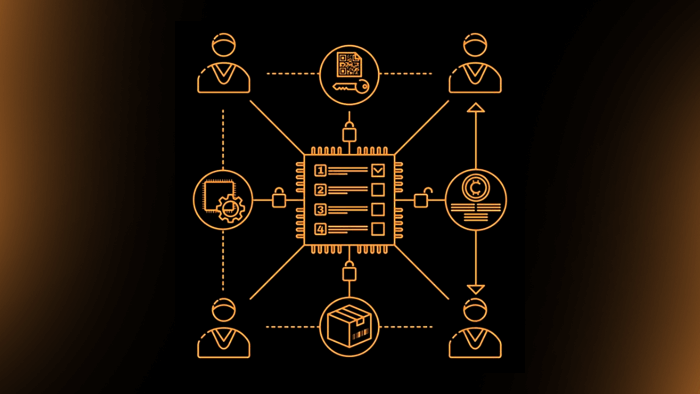
Many people believe that smart contracts are a new concept and were invented with the Ethereum Blockchain Platform. But smart contracts date back to 1996 when computer scientist Nick Szabo coined the term “smart contracts” and explained them as:
I call these new contracts “smart”, because they are far more functional than their inanimate paper-based ancestors. No use of artificial intelligence is implied. A smart contract is a set of promises specified in digital form, including protocols within which the parties perform on these promises.
His work later inspired other scientists and researchers, as well as Vitalik, who developed Ethereum.
Before we go deeper into the creation and deployment of the Ethereum smart contract, it is essential to understand the Ethereum platform and how it works.
- Ethereum, as a Blockchain Platform for developing decentralized applications
- How Ethereum Blockchain Platform executes Smart Contracts?
- What is a Smart Contract?
- What is Solidity?
- Steps to develop an Ethereum Smart Contract
- Steps to test an Ethereum smart contract
- Tools and Technologies required for implementing Ethereum Smart Contracts
Ethereum, as a Blockchain Platform for developing decentralized applications
Blockchain platforms allow developers to create and run smart contracts. Ethereum is also one of the blockchain platforms that can execute arbitrary code so that you can perform any program on Ethereum.
The Ethereum Blockchain is a potential distributed infrastructure that facilitates you to complete projects using smart contracts.
- Create your cryptocurrencies
Ethereum, you can create a tradable token that you can use as a new currency. Tokens created with the Ethereum platform use a standard coin API to be compatible with any Ethereum blockchain wallet. - Develop virtual organizations
You can write a smart contract to build a blockchain-based organization. You can then add more people to your organization and enable voting rules. Members of your organization can vote and if it reaches the required number of votes, the smart contract executes automatically. - Build dApps
Ethereum allows developers to develop secure and fault-tolerant decentralized apps that eliminate intermediaries and offer transparency. - Raise funds
You can also use Ethereum smart contracts for fundraising. With Ethereum, you can write a smart contract and a deadline. In case you fail to accomplish the goal, all donations will automatically be reimbursed to donors without disputes or commissions.
How Ethereum Blockchain Platform executes Smart Contracts
Before discussing how to create a smart contract on the Ethereum platform, you need to understand the Ethereum blockchain and how it runs smart contracts.
So, let’s understand the execution environment first.
Ethereum Virtual Machine (EVM)
The purpose of EVM is to serve as a runtime environment for smart contracts built on Ethereum. Consider it as a global supercomputer that executes all the smart contracts.
As the name indicates, Ethereum Virtual Machine is not physical but a virtual machine. The functionality of EVM is restricted to virtual machines; for example, it cannot make delayed calls on the internet or produce random numbers. Therefore, it is considered a simple state machine. Writing programs in assembly language do not make any sense, so, Ethereum required a programming language for the EVM.
Gas
In the Ethereum Virtual Machine, gas is a measurement unit used for assigning fees to each transaction with a smart contract. Each computation happening in the EVM needs some amount of gas. The more complex the computation is, the more the gas is required to run the smart contracts.
Transaction fee = Total gas used*gas price
Solidity
Solidity is a smart contract programming language on Ethereum. Developed on the top of the EVM, it is similar to the object-oriented programming language that uses class and methods. It allows you to perform arbitrary computations, but it is used to send and receive tokens and store states. When it comes to syntax, Solidity is greatly influenced by C++, Python, and Javascript so that developers can understand its syntax quickly.
It would be best to have a good understanding of Solidity programming language to efficiently write an Ethereum Smart Contract.
What is a Smart Contract?
Smart Contracts are the business logic or a protocol according to which all the transactions on a Blockchain happen. The smart contract’s general objective is to satisfy common contractual conditions like creating its own token on ethereum. We need to develop smart contracts according to which all the calculations on our token would happen.
It is a stand-alone script written in Solidity and compiled into JSON and deployed to a particular address on the blockchain. Just like we can call a URL endpoint of a RESTful API to run some logic through an HttpRequest, we can execute deployed smart contract similarly at a particular address by entering the accurate data along with Ethereum to call the compiled and deployed Solidity function.
Smart contracts are deployed to the decentralized database for a fee proportional to the containing code’s storage size. It can also be defined as a collection of code stored in the blockchain network, defining conditions to which all parties within the contract should agree.
We will be sharing the example of Ethereum smart contract creation using the Solidity programming language. So, it is first essential to understand what is Solidity.
What is Solidity?
Solidity is a Javascript-like language developed specifically for creating smart contracts. It is typed statically and supports libraries, inheritance and complex user-defined types.
Solidity compiler converts code into EVM bytecode which is sent to the Ethereum network as a deployment transaction.
Here’s a step-by-step guide to creating and deploying Ethereum Smart Contracts with Solidity
Installing Prerequisites
Meta-mask Chrome Extension
MetaMask acts both as an Ethereum browser and a wallet. It allows you to interact with smart contracts and dApps on the web without downloading the blockchain or installing any software. You only need to add MetaMask as a Chrome Extension, create a wallet and submit Ether.
Though MetaMask is currently available for Google Chrome browser, it is expected to launch for Firefox too in the coming years.
Download MetaMask chrome extension before you start writing smart contracts.
Once it is downloaded and added as a Chrome extension, you can either import an already created wallet or create a new wallet. You must have some ethers in your Ethereum wallet to deploy Ethereum smart contract on the network.
Steps to develop an Ethereum Smart Contract
Step 1: Create a wallet at meta-mask
Install MetaMask in your Chrome browser and enable it. Once it is installed, click on its icon on the top right of the browser page. Clicking on it will open it in a new tab of the browser.
Click on “Create Wallet” and agree to the terms and conditions by clicking “I agree” to proceed further. It will ask you to create a password.
After you create a password, it will send you a secret backup phrase used for backing up and restoring the account. Do not disclose it or share it with someone, as this phrase can take away your Ethers.
You should either write this phrase on a piece of paper securely or store it safely on an external encrypted hard drive where no one could find it.
The next step is to ensure that you are in the “Main Ethereum Network.” If you find a checkmark next to “Main Ethereum Network”, you are in the right place.
Step 2: Select any one test network
You might also find the following test networks in your MetaMask wallet:
- Robsten Test Network
- Kovan Test Network
- Rinkeby Test Network
- Goerli Test Network
The above networks are for testing purposes only; note that these networks’ ethers have no real value.
Step 3: Add some dummy Ethers to your wallet
In case you want to test the smart contract, you must have some dummy ethers in your MetaMask wallet.
For example, if you want to test a contract using the Robsten test network, select it and you will find 0 ETH as the initial balance in your account.
To add dummy ethers, click on the “Deposit” and “Get Ether” buttons under Test Faucet.
LeewayHertz’s Ethereum Smart Contract Development
To proceed, you need to click “request one ether from the faucet,” and 1 ETH will be added to your wallet. You can add as many Ethers you want to the test network.
For example, I have added 1 ETH in this scenario.
Once the dummy ethers are added to the wallet, you can start writing smart contracts on the Remix Browser IDE in the Solidity programming language.
Step 4: Use editor remix to write the smart contract in Solidity
We will use Remix Browser IDE to write our Solidity code. The remix is the best option for writing smart contracts as it comes with a handful of features and offers a comprehensive development experience.
It is usually used for writing smaller-sized contracts. Remix’s features include:
- Warnings like gas cost, unsafe code, checks for overlapping variable names, whether functions can be constant or not
- Syntax and error highlighting
- Functions with injected Web3 objects
- Static analysis
- Integrated debugger
- Integrated testing and deployment environment
- Deploy directly to Mist or MetaMask
Let’s start writing smart contract code by visiting https://remix.ethereum.org.
Step 5: Create a .sol extension file
Open Remix Browser and click on the plus icon on the top left side, next to the browser to create a .sol extension file.
Step 6: A sample smart contract code to create ERC20 tokens
ERC20.sol is a standard template for ERC20 tokens.
pragma solidity ^0.4.0; import "./ERC20.sol"; contract myToken is ERC20{ mapping(address =>uint256) public amount; uint256 totalAmount; string tokenName; string tokenSymbol; uint256 decimal; constructor() public{ totalAmount = 10000 * 10**18; amount[msg.sender]=totalAmount; tokenName="Mytoken"; tokenSymbol="Mytoken"; decimal=18; } function totalSupply() public view returns(uint256){ return totalAmount; } function balanceOf(address to_who) public view returns(uint256){ return amount[to_who]; } function transfer(address to_a,uint256 _value) public returns(bool){ require(_value<=amount[msg.sender]); amount[msg.sender]=amount[msg.sender]-_value; amount[to_a]=amount[to_a]+_value; return true; } }
Select a version of the compiler from Remix to compile the solidity Ethereum smart contract code.
Step 7: Deploy your contract
Deploy the smart contract at the Ethereum test network by pressing the deploy button at the Remix window’s right-hand side.
Wait until the transaction is complete.
After the transaction commits successfully, the address of the smart contract would be visible at the right-hand side of the remix
window.
At first, all the ERC20 tokens will be stored in the wallet of a user who is deploying the smart contract.
To check the tokens in your wallet, go to the metamask window, click add tokens, enter the smart contract address and click ok. You would be able to see the number of tokens there.
Steps to test an Ethereum smart contract
- Try to run all your smart contract methods like transfer, total supply, and balance(in the above smart contract example). These methods are present at the right-hand side of the remix window and you can run all the processes from there itself.
- Try to transfer some tokens to other ethereum wallet addresses and then check the balance of that address by calling the balance method.
- Try to get total supply by running the total supply method.
Steps to deploy Ethereum Smart Contracts
- To make your smart contract live, switch to the main ethereum network at metamask
- Add some real ethers.
- Now again, deploy your smart contract using remix as mentioned in the above steps.
- When a smart contract is deployed successfully, visit http://www.etherscan.io and search your smart contract address there. Select your smart contract.
- Now you need to verify your smart contract here, click “verify the contract.”
- Copy your smart contract code and paste it at Etherscan. Select the same compiler version that you selected at remix to compile your code.
- Check “optimization” to Yes, if you had selected optimization at remix; otherwise, select No.
- Click Verify.
- It will take a few minutes and your smart contract will be live if no issue occurs.
- You can now run your smart contract methods at Etherscan.
Tools and Technologies required for implementing Ethereum Smart Contracts
- Truffle
t is an Ethereum development framework that allows developers to write and test smart contracts. Written in JavaScript, Truffle contains a compiler for the Solidity programming language. Truffle Contract is a JavaScript library that allows importing of compiled smart contracts. - Web3.js
It is an Ethereum JavaScript API that interacts with the Ethereum network via RPC calls. - Visual Studio Code
A functional code editor. - Ganache CLI
It is an Ethereum remote procedure call client within the Truffle framework that is also known as TestRPC. - Parity
It is a secure and fast Ethereum client for handling Ethereum accounts and tokens. - Node.js
It is a javascript runtime environment used for server-side programming. Node.js is required to test the Ethereum smart contract’s functionality while ensuring its secure and proper operation. You need to install a package manager, for example, Yarn along with Node.js.
We have a team of smart contract developers who ensure to develop secure and efficient smart contracts for various purposes, including crowdfunding, bidding, permissioning, and dApps. In case, you are looking to deploy smart contracts for your business operations, consult us and discuss your requirements.
Start a conversation by filling the form
All information will be kept confidential.
Insights
How to create ERC-20 token on Ethereum network?
ERC20 tokens, executed as smart contracts on the Ethereum Virtual Machine , define rules of interaction and purchase with other tokens.
ERC20 vs ERC721 vs ERC1155
ERC20, ERC721, and ERC1155 are the prevalent token standards as approved by the Ethereum community.
How to develop NFT Marketplace on Ethereum?
With the help of NFT marketplace developed on Ethereum blockchain, you can leverage open transaction history for ownership verification.